Commission Balance Widget
Admin: Web Office Admin Page: Code Customization > Custom Widgets URL: {client_ID}.admin.directscale.com/#/Widgets
This widget displays the associate's commission balance available in the payment provider (merchant) configured in your system. This example uses i-Payout as the payment provider, but it can be customized to work with your chosen payment provider.
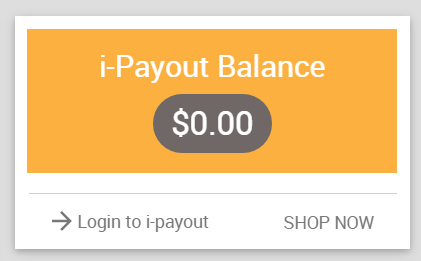
You can use the following code as is or as a starting point for something even better!
<script type="text/ng-template" id="Ipayoutwallet.tpl.html">
<div>
<!-- Front side of flipper. -->
<div class="card">
<div id="Ipayoutwallet">
<div id="IpayoutwalletBalance" class="p-10">
<div class="card-header bgm-color4 c-white">
<h2 class="f-s-24 text-center" translate>ipayoutwallet_balance</h2>
<div class="ipayoutwalletpricecenter">
<div class="ipayoutwalletprice bgm-color3">
<span ng-bind="ipayoutwalletBalance | currency"></span>
</div>
</div>
</div>
<div class="card-body p-b-15 p-r-15">
<div class="row border_top">
<div class="p-t-10">
<div class="login-btn" ng-if="widgetCtrl.HasipayoutwalletAccount">
<a target="_blank" href="@GetSsoUrl("https://examplecompany.globalewallet.com/public/single-sign-on.aspx?token={DirectScale:Token}")" class="card-footer card-footer--link">
<svg class="card-footer__icon" xmlns="http://www.w3.org/2000/svg" xmlns:xlink="http://www.w3.org/1999/xlink" version="1.1"
width="24" height="24" viewBox="0 0 24 24">
<path d="M4,11V13H16L10.5,18.5L11.92,19.92L19.84,12L11.92,4.08L10.5,5.5L16,11H4Z" />
</svg>
<span id="loginipayoutwallet" translate>Login_ipayoutwallet</span>
</a>
</div>
<div class="shop-btn">
<a ui-sref="OrderProductList" class="card-footer card-footer--link">
<span id="shopnow" translate class="p-t-3">shop_now</span>
</a>
</div>
</div>
</div>
</div>
</div>
</div>
</div>
<div class="spinner" ng-class="{'spinner--active': widgetCtrl.loading}"></div>
</div>
</script>
<dsw-ipayoutwallet user-id="userId" user="user"></dsw-ipayoutwallet>
#Ipayoutwallet {
.ipayoutwalletpricecenter {
display: flex;
justify-content: center;
align-items: center;
}
.ipayoutwalletprice {
display: inline-block;
border-color: red;
border: 2px black;
font-size: 26px;
text-align: center;
margin-top: 10px;
background-color: #337ab7;
border-radius: 30px;
padding: 5px 15px;
}
.p-t-3 {
padding-top: 3px;
}
.login-btn {
width: 64%;
position: relative;
min-height: 1px;
padding-left: 15px;
padding-right: 15px;
float: left;
}
.shop-btn {
width: 36%;
position: relative;
min-height: 1px;
padding-left: 15px;
padding-right: 15px;
float: left;
}
.card-footer--link {
padding: 0px;
border: none;
}
.card-footer--link .card-footer__icon {
margin-right: 0px;
}
.border_top {
border-top: 1px solid #cfcfcf;
}
.card-body {
padding: 16px 16px 0px 16px;
}
.p-b-15 {
padding-bottom: 0px !important;
}
}
module.directive('dswIpayoutwallet', [function () {
return {
templateUrl: 'Ipayoutwallet.tpl.html',
scope: {
userId: '=',
user: '='
},
restrict: 'E',
controllerAs: 'widgetCtrl',
controller: ['$scope', '$RestService', 'UserService', '$translate', 'flipperService', 'Notification', function ($scope, $RestService, UserService, $translate, flipperService, Notification) {
var ctrl = this;
ctrl.loading = true;
ctrl.getipayoutwalletBalance = function () {
ctrl.loading = true;
var request = {
associateId: $scope.user.CustomerId,
merchantId: 112
};
// merchantId for i-payout:112
$RestService.DynamicV2PostMethod('api/Payment/GetAssociateMerchantBalance', request).then(function (result) {
result = result.data;
ctrl.loading = false;
ctrl.HasipayoutwalletAccount = false;
if (parseInt(result && result.Status, 10) === 0) {
$scope.ipayoutwalletBalance = result.Data.Balance >= 0 ? result.Data.Balance : 0;
if (result.Data.Balance >= 0) {
ctrl.HasipayoutwalletAccount = true;
}
}
}).catch(function (error) {
error = error.data;
ctrl.loading = false;
});
};
ctrl.getipayoutwalletBalance();
}]
};
}]);
SSO Link
In the HTML code example, the link in the widget footer is shown as an SSO link:
<a target="_blank" href="@GetSsoUrl("https://examplecompany.globalewallet.com/public/single-sign-on.aspx?token={DirectScale:Token}")" class="card-footer card-footer--link">
To read more about SSO linking in the DirectScale Platform, see: Inbound/Outbound Single Sign-On (SSO)
Entering MerchantID
In the JavaScript code example, you can see the following snippet:
ctrl.getipayoutwalletBalance = function () {
ctrl.loading = true;
var request = {
associateId: $scope.user.CustomerId,
merchantId: 112
};
// merchantId for i-payout:112
In this function, you enter the merchantId
of your payment provider.
If you don't know your money out payment provider's Merchant ID, you can find it here: Money In/Out MerchantIDs.
Adding String Keys
When using the preceding code, the custom widget will display like the following image when added to Web Office:
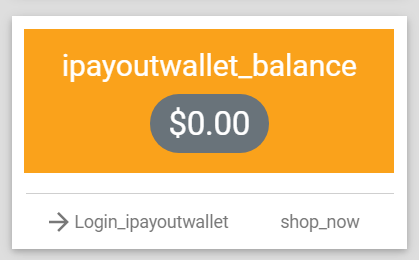
You'll notice that the text elements aren't ideal. That's because, in the HTML, string keys were added in place of static text to allow for dynamic translation to other languages.
<h2 class="f-s-24 text-center" translate>ipayoutwallet_balance</h2>
Whatever defined string keys need to be added in the Web Office Admin Localization page for the widget to display correctly:
For example:
- Key:
ipayoutwallet_balance
- Example Text Value: "i-Payout Balance"
- Key:
Login_ipayoutwallet
- Example Text Value: "Login to i-payout")
- Key:
shop_now
- Example Text Value: "SHOP NOW"
Updated about 2 months ago